1) Method overloading is a means of allowing classes to handle different types of data in a uniform manner. Multiple functions with the same name exist at the same time, with different parameter numbers/types. Overloading is a manifestation of polymorphism in a class.
2) Java's method overloading is that you can create multiple methods in a class, they have the same name, but with different parameters and different definitions. When calling a method, the number of different parameters and the type of the parameter passed to them are given to their different parameter numbers and parameter types to determine the number of different parameters and parameter types to determine which method to use. This is polymorphism.
3) When overloading, the method name is the same, but the parameter type and number are different, and the return value types can be the same or different. The return type cannot be used as a distinguishing criterion for overloaded functions.
example
Package c04.answer;//This is the package name
/ / This is the first programming method of this program, first create a Dog class instance in the main method, then in the Dog
The constructor of the class uses the this keyword to call different bark methods. Different overloaded methods bark are distinguished according to their parameter types.
//Note: Except for the constructor, the compiler prohibits calling constructors anywhere else.
Package c04.answer;
Public class Dog {
Dog()
{
This.bark();
}
Void bark()//bark() method is overloaded method {
System.out.println("no barking!");
This.bark("female", 3.4);
}
Void bark(String m,double l)//Note: The return value of the overloaded method is the same.
{
System.out.println("a barking dog!");
This.bark(5, "China");
}
Void bark(int a, String n)// cannot distinguish overloaded methods with return values, but can only distinguish between "parameter type" and "class name" {
System.out.println("a howling dog");
}
Public staTIc void main(String[] args)
{
Dog dog = new Dog();
//dog.bark();
//dog.bark("male", "yellow");
//dog.bark(5, "China");
1) Polymorphism between the parent class and the child class, redefining the function of the parent class. If a method is defined in a subclass with the same name and parameters as its parent class, we say that the method is overridden. In Java, subclasses can inherit methods from the parent class without having to rewrite the same method. But sometimes subclasses don't want to inherit the methods of the parent class intact, but want to make certain modifications, which requires a rewrite of the method. Method rewriting is also called method coverage.
2) If the method in the subclass has the same method name, return type, and parameter list as one of the methods in the parent class, the new method will overwrite the original method. For the original method in the parent class, you can use the super keyword, which refers to the parent class of the current class.
3) The access modification permission of the subclass function cannot be less than that of the parent class;
example
Public class Base
{
Void test(int i)
{
System.out.print(i);
}
Void test(byte b)
{
System.out.print(b);
}
}
Public class TestOverriding extends Base
{
Void test(int i)
{
i++;
System.out.println(i);
}
Public staTIc void main(String[]agrs)
{
Base b=new TestOverriding();
B.test(0)
B.test((byte)0)
}
}
The output at this time is 1 0, which is the result of dynamic binding at runtime.
Method Override Overriding and Overloading Overloading are different manifestations of Java polymorphism. Overriding Overriding is a manifestation of polymorphism between parent and child classes. Overloading is a manifestation of polymorphism in a class. If a method is defined in a subclass with the same name and parameters as its parent class, we say that the method is overridden. When an object of a subclass uses this method,
The definition in the subclass will be called. For it, the definition in the parent class is "masked", and if the method name and parameter type and number of the subclass are the same as the parent class, then the return type of the subclass Must be the same as the parent class; if multiple methods with the same name are defined in a class, they may have different parameter numbers or different parameter types, which is called overload of the method. The method of Overloaded is to change the type of the return value. In other words, the overloaded return value types can be the same or different.
1) The compiler checks the type and method name of the object declaration to get all candidate methods. Try to comment out the test of the Base class in the above example, and then compile it will not pass.
2) Overload decision: The compiler checks the parameter type of the method call, and selects the only one from the candidate method (there will be an implicit type conversion). If the compiler finds more than one or fails to find it, the compiler will complain. Try to comment out the test(byte b) of the Base class in the above example, and the result is 1 1 .
3) If the method type is priavte staTIc final and java is statically compiled, the compiler will know exactly which method to call.
4) When the program runs and uses dynamic binding to call a method, then the virtual machine must call the method version that matches the actual type of the object. In the example, the actual type pointed to by b is TestOverriding, so b.test(0) calls the test of the subclass. However, the subclass does not override test(byte b), so b.test((byte)0) calls the parent class's test(byte b). If the parent class's (byte b) is commented out, the second type of implicit type is converted to int, and finally the subclass's test(int i) is called.
Yidashun offer replacement Laptop Charger for LS, the OEM LS power Adapter 100% compatible works for your LS notebook PC, meets or exceeds the original adapter!
Our adapter are with smart IC to protect your laptop with over current protection, over load protection, short circuit protection, over heat protection. If your family LS adapter is broken, please kindly check your laptop model and confirm the model you need, you can choose OEM replacement models for recharge your laptop! Yidashun offer wholesale factory direct prices at 1 year warranty!
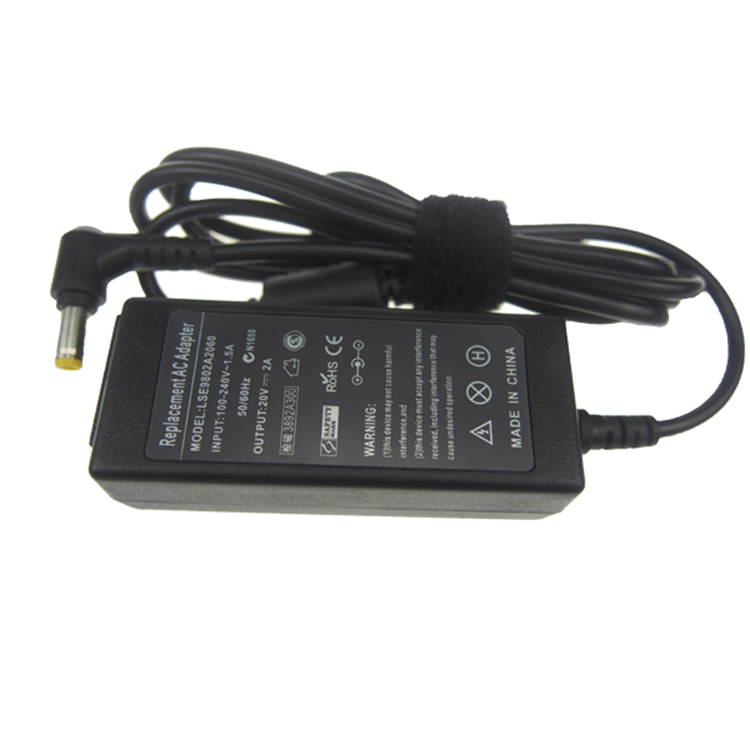
LS Laptop Charger,LS Computer Charger,LS Notebook Charger,LS Laptop Battery Charger
Shenzhen Yidashun Technology Co., Ltd. , https://www.ydsadapter.com